The biggest source of nice ideas on swing UI I got from JGoodies. The Author of Jgoodies libraries, Karsten Lentzsch, I could proudly call my inspirational guru. First time I visited jgoodies.com site was 5 years back and used it in my first Java Applet. Later I reused all that code in desktop application. I liked its look, layout manager, form builders and simplicity, I also saw clear deep understanding of UI design and usability issues.
My personal experience
Now I'm using all his libraries: jgoodies-forms, jgoodies-looks, jgoodies-validation and jgoodies-binding. He was one of the first people who showed and said how swing application can and should look and behave, that was before anyone started to talk about these problems in loud.
Six years ago, once again I went on freelance. Major focus for my service was and still is on Java Web applications for business. As always happens from time to time I had to do some swing programming. I and my company (now I'm running small software development business) did 4 applications on swing, all of them was based on jgoodies. All these applications are functioning perfectly, customers are happy, and have best opinion about desktop software developed on swing. All of them like application's look, usability, easy of use and performance. Looks like great success story.
Now Karsten Lentzsch, author of JGoodies is on JCP-295 Beans Binding and JCP-296 Swing Application Framework expert group list. I hope that will come to great specifications and products.
Some points
IMHO good code is a code which is easy to read, understand and follows natural way of thinking. It has be easy to understand by the person who will come after me and will have to extend, modify or support it. Another important point that UI should be consistent and elegant.
As wiki or blog editor does not care about HTML, CSS, HTTP or all other technical stuff you don't have to care about SWING components. In case you know what you are doing you can mess with swing, but you should do that only where it's needed, not everywhere. So we need some abstraction layer, layer which seats between end user and swing components.
How do we use swing components? Simple answer is inefficient! Every time I have to add a new record such as Customer, Product or whatever, we are dealing with JTextField, JDialog, JButtom, JAction, different action listeners. But this way is too deep, takes too much time and is hugely inefficient, everything I want is a common add, edit or delete form, where I have a list of required and optional fields and Ok, Cancel buttons. It would be nice to have some help or hints and validation of entered data.
And at last there is some Domain Specific Languages, this is where I'm leading. I'm not going to go through Domain language definition, everything is on wikipedia domain specific languages. This Domain Specific Language, or UI abstraction layer should hide unneeded details and hide complexity, but without introducing new limitations. I mean, if I need a deeper control and still want to deal with swing controls, I still can do that and don't want to have another Domain language which I have different syntax to learn and maintain.
Just to tease you here is a window's screenshot from one of my application. All these windows are consistent across all application, they follow common UI guidelines. There is one small but, I have to remove these classes from my old applications, do some little cleaning, update them a little bit and make them available as open source package.
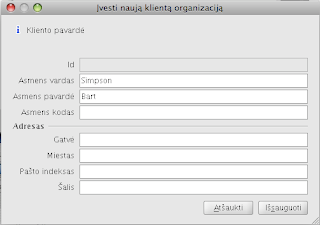
In order to collect all requirements and update I need some opinion from outside.
What we can expect to get at the end:
- less lines of code - better productivity, less bugs
- source code have to be easy to read, understand and maintain
- UI - must be consistent and with no surprises
- UI - should be nice and pleasant to work with
- software developer have to be able to change UI as he likes, UI does not have to be forced by framework
- It does not have to meet everyones expectation. Bloated library is the worst thing can be.